Super learner
This user is a Super Learner. To become a Super Learner, you need to reach Level 8.
2-D histogram: Density matrix values in their correct position.
Hi Viktor, you indicated in the lesson on Histograms in NumPy (part 2) that in the density array returned by the np.histogram2d() function there is a slight rotation because the rows of said array correspond to the X bins and the columns to the Y bins. In other words, the rows are swapped with the columns with respect to a visual representation.
Using Python and Numpy features I wrote a line of code that repositions the values of the density matrix so that it corresponds directly, without rotations, with its graphical representation in the X, Y coordinate plane.
The code is as follows,
np.array(list(map(lambda row: row[::-1], dm))).T
dm, is de density array.
dm = np.histogram2d(matrix_A[0], matrix_A[1], bins=4)[0]
And I ask you about it if you agree with me that the resulting matrix in this case is the density array that fully corresponds to its graphical representation in the X, Y plane and that this matrix is practically also giving us the visual representation of the corresponding 2-D histogram
3 answers ( 0 marked as helpful)
Super learner
This user is a Super Learner. To become a Super Learner, you need to reach Level 8.
I added some graphs and, at least for the lesson example, I see that the graph clearly looks like the repositioned value density matrix:
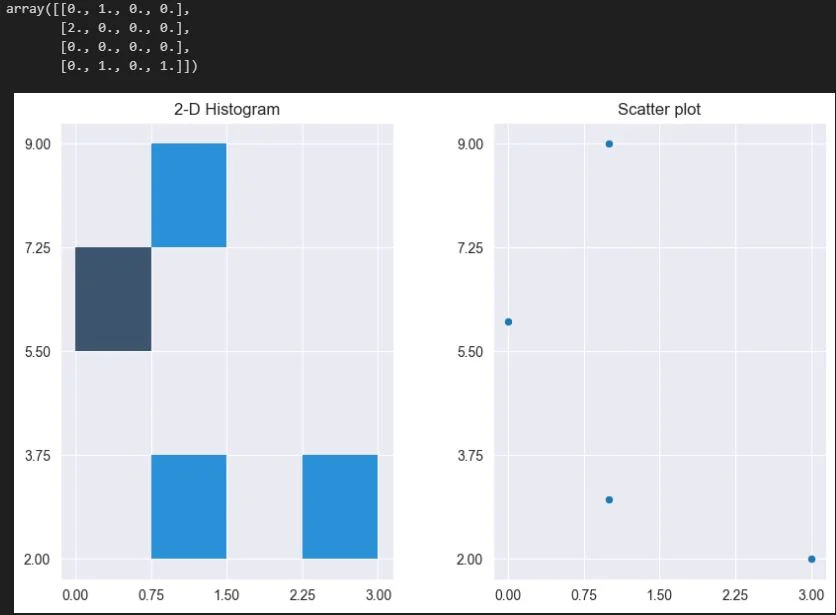
Super learner
This user is a Super Learner. To become a Super Learner, you need to reach Level 8.
Reading about different alternatives to order elements in an array in decreasing order, I found the np.flip() function, which just reverses the order of the elements in an array on a given axis preserving the shape of the array.
Function that simplifies the task of rearranging the elements of the original density array of np.histogram2d() so that said array allows a direct reading with the histogram2d graph or the scatter plot.
So the code, mentioned above, now using np.flip would be much simpler:
np.flip(dm, axis=1).T
dm = np.histogram2d(matrix_A[0], matrix_A[1], bins=4)[0]
Function that simplifies the task of rearranging the elements of the original density array of np.histogram2d() so that said array allows a direct reading with the histogram2d graph or the scatter plot.
So the code, mentioned above, now using np.flip would be much simpler:
np.flip(dm, axis=1).T
dm = np.histogram2d(matrix_A[0], matrix_A[1], bins=4)[0]
Super learner
This user is a Super Learner. To become a Super Learner, you need to reach Level 8.
Reviewing the indexing of numpy arrays, I realized that there is another way to rearrange the values of the density matrix. I copy it here because I found it very interesting and it also shows the great potential of indexing:
dm[:,::-1].T
dm[:,::-1].T
dm = np.histogram2d(matrix_A[0], matrix_A[1], bins=4)[0]
By properly handling indexing, the np.flip() function becomes practically unnecessary:
np.flip(array); equivalent to array[::-1,::-1]
np.flip(array, axis=1); equivalent to array[:,::-1]
np.flip(array, axis=0); equivalent to array[::-1,:]
Likewise, in the case where we want to sort numerical values in descending order, we can not only use
-np.sort(-array, axis=None)
but also any of the following lines:
np.flip(np.sort(array, axis=None))
np.sort(array, axis=None)[::-1]